An array is a sequential collection of variables of same data type which can be accessed using an integer as index, that generally starts from 0. It stores data elements in a continuous memory location. Each element can be individually referenced with its respective index.
1-Dimensional array: It is a linear array that stores elements in a sequential order. Let us try to demonstrate this with an example: Let us say we have to store integers 2, 3, 5, 4, 6, 7. We can store it in an array of integer data type. The way to do it is:
Syntax and Declaration
Basic Declaration: Show how to declare an array in C.
<datatype> <variablename><[sizeofarray]>;
int numbers[5]; // Declares an array of 5 integers
Initialization: Demonstrate how to initialize an array.
int numbers[5] = {1, 2, 3, 4, 5}; // Initializes an array with values
Here ‘number‘ is the name of array and 5 is the size. It is necessary to define the size array at compile time.
Let’s understand it
dataType nameOfTheArray [ ] = {elements of array };
int Arr [ 6] = { 2, 3, 5, 4, 6, 7 };
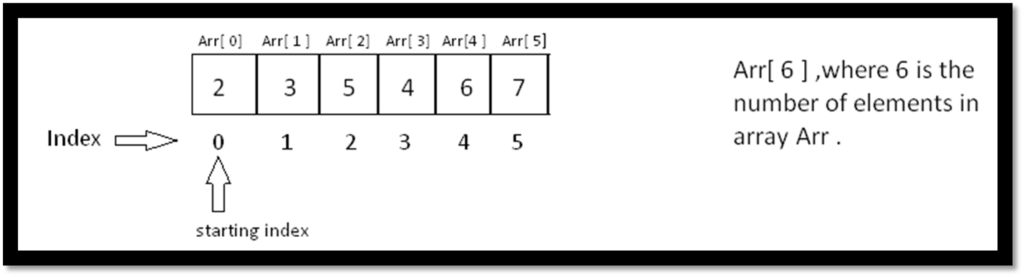
Since, the indexing of an array starts from 0, if the 4th element needs to be accessed, Arr [ 3 ] will give you the value of the 4th element. Similarly, nth element can be accessed by Arr[n-1].
Accessing Array Elements
Indexing: Array indexing starts at 0. Show how to access and modify elements.
printf("%d", numbers[0]); // Accesses the first element
numbers[1] = 10; // Modifies the second element
Definition of Array
#include<stdio.h>
int main(void)
{
int a[5];
a[0]=9;
a[1]=10;
a[2]=14;
a[3]=76;
a[4]=34;
for(int i=0; i<5; i++)
{
printf("%d",a[i]);
}
}
#include<stdio.h>
int main(void)
{
int a[5]={9,4,22,18,17};
for(int i=0; i<5; i++)
{
printf("%d",a[i]);
}
}
Looping through Arrays
int a[5]={5,6,8,2,4};
for (int i = 0; i < 5; i++) {
printf("%d ", a[i]); // Prints each element
}
Example:
#include<stdio.h>
int main(void)
{
int a[5];
printf("Enter the 5 values: ");
for(int i=0; i<5; i++)
{
scanf("%d",&a[i]);
}
for(i=0; i<5; i++)
{
printf("\nThe Values are: %d",a[i]);
}
}