A while
loop in C is a control flow statement that allows you to repeatedly execute a block of code as long as a specified condition remains true. It’s one of the fundamental looping structures in C and is great for scenarios where the number of iterations is not known beforehand. The while loop can be thought of as a repeating if statement.
How It Works
Here’s a simple breakdown of how a while
loop operates:
- Condition Check: Before each iteration of the loop, the condition specified in the
while
statement is evaluated. - Code Execution: If the condition is true, the code inside the loop’s block is executed.
- Repeat: After executing the code block, the condition is checked again. If it’s still true, the loop repeats; otherwise, it exits.
- Exit: When the condition becomes false, the loop terminates, and the program continues with the code that follows the loop.
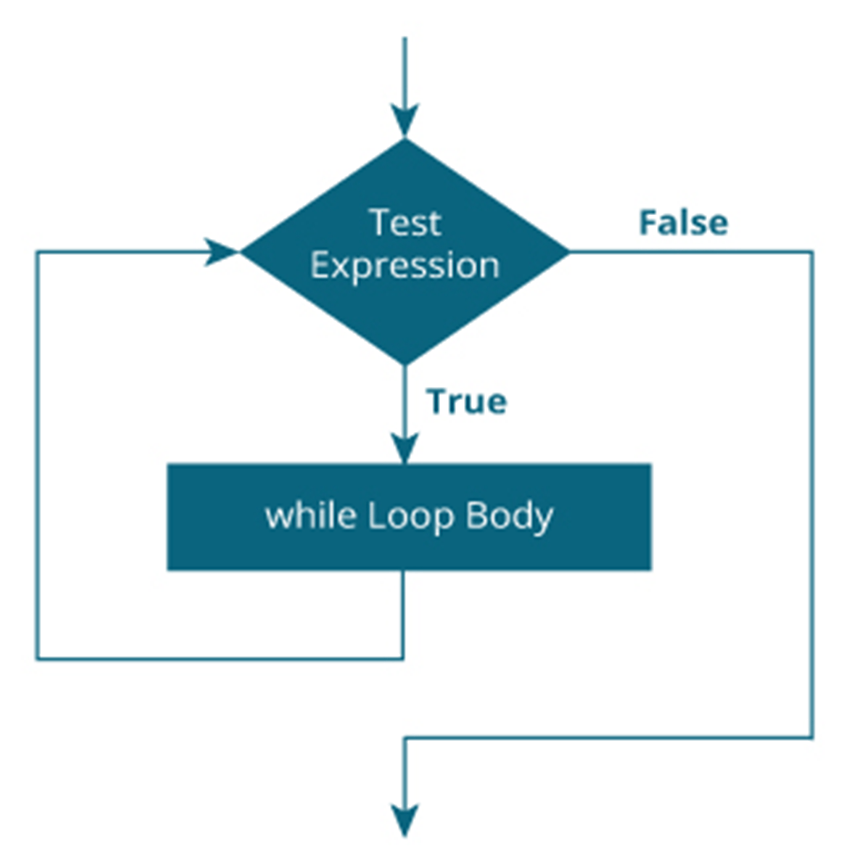
Syntax
while (testExpression)
{
// statements inside the body of the loop
}
Example: while loop
#copycode
#include <stdio.h>
int main() {
int i = 1; // Initialize the loop control variable
while (i <= 5) { // Condition: Continue looping while i is less than or equal to 5
printf("%d\n", i); // Print the current value of i
i++; // Increment i by 1
}
return 0;
}
In this example:
- Initialization:
i
is set to 1 before the loop starts. - Condition: The loop continues as long as
i
is less than or equal to 5. - Body: Inside the loop, it prints the value of
i
and then incrementsi
by 1. - Termination: When
i
becomes 6, the conditioni <= 5
is false, so the loop exits, and the program ends.
Common Uses
- Reading Input: Keep reading user input until a certain condition is met (e.g., until the user enters a specific value).
- Processing Data: Continuously process items in a data stream or collection until the end is reached.
- Repeating Actions: Perform an action repeatedly until a task is completed or a condition changes.
Key Points
- Infinite Loops: Be cautious with
while
loops. If the condition never becomes false, the loop will run indefinitely. This is known as an infinite loop and can cause your program to hang or crash. - Updating Variables: Ensure that the loop’s condition will eventually become false by modifying the loop control variables within the loop.