In C, string is stored in an array of characters. Each character in a string occupies one location in an array. The null character ‘\0’ is put after the last character. This is done so that program can tell when the end of the string has been reached.
char c[] = “any name”;
When the compiler encounters a sequence of characters enclosed in the double quotation marks, it appends a null character \0 at the end by default.
Declaration
char s[5]; //Here, we have declared a string of 5 characters.
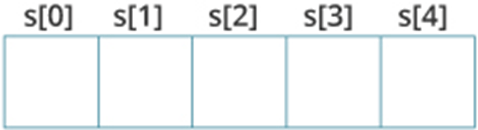
Initialization
char c[] = “abcd”;
char c[50] = “abcd”;
char c[] = {‘a’, ‘b’, ‘c’, ‘d’, ‘\0’};
char c[5] = {‘a’, ‘b’, ‘c’, ‘d’, ‘\0’};
Example:
#include <stdio.h>
void main ()
{
char a[7] = { ‘e’, ‘x’, ‘a’, ‘m’, ‘p’, ‘l’, ’e’ };
char b[10];
printf (“Enter the value for B”);
scanf("%s",b);
printf ("A Output: %s\n", a);
printf ("B Output: %s\n", b);
}
String Functions
- strlen(“name of string”)
- strcpy( dest, source)
- strcmp( string1, string2 )
- strstr( str1, str2 )
String copy and length
#include <stdio.h>
#include <string.h>
Int main ()
{
char a[7] = { 'n', 'i', 's', 'h', 'a', 'n' };
char b[10]; int c; //scanf("%s",a);
printf ("A Output: %s\n", a);
strcpy(b, a); c=strlen(b);
printf ("B Output: %s\n", b);
printf ("B Output: %d\n", c);
return 0;
}
strcmp( str1 , str2 );
This function is used to compare two strings “str1” and “str2”. this function returns zero(“0”) if the two compared strings are equal, else some none zero integer.
Return value
– if Return value if < 0 then it indicates str1 is less than str2
– if Return value if > 0 then it indicates str2 is less than str1
– if Return value if = 0 then it indicates str1 is equal to str2
Normal approach for comparing two strings is
Example:
Program to identify two strings are equal or not.
#include <stdio.h>
#include <string.h>
void main ()
{
char a[10];
char b[10];
int c;
printf ("\nEnter the value for a: ");
scanf("%s",a);
printf ("\nEnter the value for b: ");
scanf("%s",b);
if(strcmp(b, a)==0)
{
printf ("Strings are equal");
}
else
{
printf ("Strings are not equal");
}
}
Output:
Enter the value of a: example
Enter the value of b: example
Strings are equal.
Strstr()
#include<stdio.h>
#include<string.h>
int main ()
{
char str1[55] ="This is a test string for testing";
char str2[20]="test";
char *p;
p = strstr (str1, str2);
if(p)
{
printf("string found\n" );
}
else printf("string not found\n" );
return 0;
}
Output:
String found